Graph Representation using Adjacency Matrix - The Coding Shala
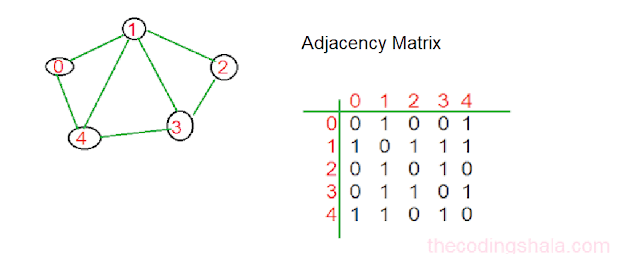
Home >> Data Structures >> Graph Representation using Adjacency Matrix Graph Representation using Adjacency Matrix In this post, we will see how to represent a graph using the Adjacency Matrix. Generally, the Adjacency matrix is used to check if there is any edge available between two vertices or not. In the Adjacency matrix, we take a 2D array of size v*V, where V is the number of vertices in a graph and array[i][j] = 1 indicates that there is an edge between vertex i and j. The following example explains the Adjacency matrix of a graph: Graph Representation using Adjacency Matrix Java Program We have given the number of vertices 'v' and edges 'E' of a bidirectional graph. Our task is to build a graph through the adjacency matrix and print it. Example 1: Input: 5 7 0 1 0 4 1 2 1 3 1 4 2 3 3 4 Output: 0 1 0 0 1 1 0 1 1 1 0 1 0 1 0 0 1 1 0 1 1 1 0 1 0 Java Code: import java.util.* ; cl