Java Encapsulation - The Coding Shala
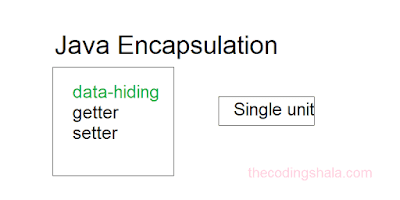
Home >> Learn Java >> Java Encapsulation Java Encapsulation Java Encapsulation is a process of wrapping code and data together into a single unit. We can say Encapsulation is a protective shield that prevents the data from being accessed by the code outside this shield, for example, a capsule. In encapsulation, the variables or data of a class are hidden from any other class and can be accessed only through any member function of its own class so it is also known as data-hiding. We can achieve Java Encapsulation by declaring all the variables in the class as private and writing public methods in the class to set and get the values of variables. These public methods called getter and setter methods. Using these getter and setter we can make our class read-only or write-only. Using Encapsulation we can control the data. The encapsulation class is easy to test. The Java Bean class is the example of a fully encapsulated class. Example of Java Encaps...