Java Classes and Objects - The Coding Shala
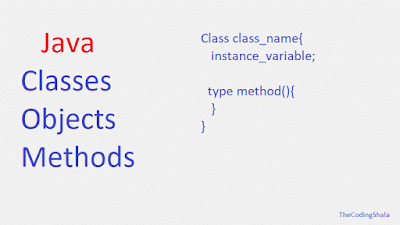
Home >> Learn Java >> Java Classes and Objects Java Classes Java classes are a structure or blueprint for which we create objects. When we create a class then it defines a new data type. This new data type is used to create objects of that type. The class keyword is used to declare a class. A class contains variables and methods. The basic structure of a class is as follows: class class_name { type instance_variable1 ; type instance_variable2 ; ... .. type instance_variableN ; type method_name ( parameters ){ //body of method } type method_name2 ( parameters ){ //body of method } ... ... type method_nameN ( parameters ){ //body of method } } The Variables, defined within a class are called instance variables. The methods and variables defined within a class are called members of the class. Note: Java classes do not need to have a main() method. If the class is the starting point of our program then the main() method